Slang v1: A reliable way to analyze Solidity code
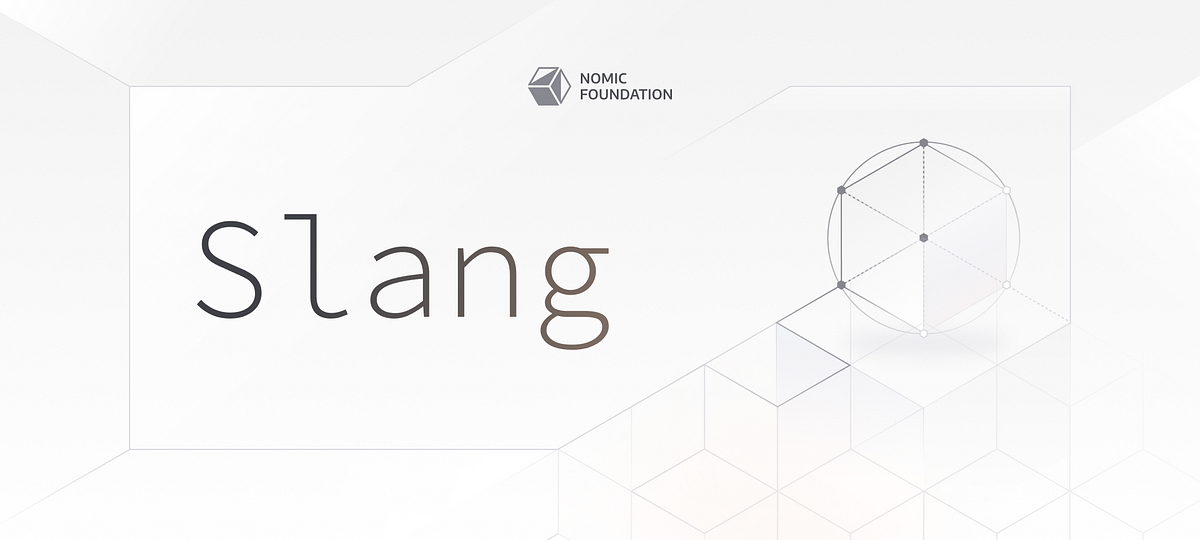
We're thrilled to share the stable release of Slang v1, after years of development and community feedback. Slang’s code analysis APIs are now production-ready and fully featured.
What’s Slang?
Slang is a modular set of compiler APIs to empower the next generation of Solidity code analysis and tooling for Ethereum developers. It’s written in Rust, and for now accessible primarily through a TypeScript API.
Building reliable tools for Solidity has always been challenging. Through Nomic Foundation’s work on building and maintaining the Hardhat suite throughout the years, we learned from first-hand experience that reliably and accurately analyzing Solidity code across its numerous versions required significant engineering effort.
That's why we built Slang as a dev tooling-first API that handles these complexities, to make it meaningfully easier for the Ethereum ecosystem to build advanced Solidity developer tools.
What can you build using Slang?
Slang is built from the ground up as a compiler API to be used by other developer tools. This gives all other Solidity tools like IDEs, formatters, linters, code verifiers, test runners, code generators, fuzzers, static code analyzers, execution simulators, block explorers, wallets, and more, a solid foundation to build on, significantly reducing the cost and difficulty of developing and maintaining these tools.
How is it different from other Solidity compilers?
Slang is designed as an API to build developer tools, while most other Solidity compilers available are designed for Solidity developers, and focus on producing byte code for deployment. What this means concretely is that Slang makes first-class citizens out of the use cases that are critical for developers of code analysis tools, starting by providing programmatic APIs as the primary interface to use the compiler.
What features are included in Slang v1?
Slang’s v1 release covers the frontend surface of the Solidity compilation APIs.
Omni-Version Support
To analyze Solidity code you’ll face the challenge of analyzing different Solidity versions. Compilers like solc handle a single version of Solidity per release, which means that to gracefully analyze multiple Solidity versions, you’ll need to have adhoc logic to use the appropriate compiler version, and handle the structural differences in the output the compiler provides across the different Solidity versions.
Slang supports 80+ versions of Solidity (from 0.4.11
to 0.8.28
) with a consistent API. No need for version-specific code paths. Write your tools once, and support all versions in one go. The output (CST, binding graph, etc.) remains stable across versions, making it perfect for version comparisons and migrations. You can now focus on building features instead of dealing with breaking changes across versions.
This has been tested on over 1,350,000 contracts from the Sanctuary dataset, with 100% accuracy for all language versions there. We are also looking into adding more chains/networks to our testing, to make sure our APIs are always up-to-date.
Error-tolerant Parser
Slang’s parser gracefully handles syntax errors, while still producing complete syntax trees. This is essential for real-world software development scenarios where code that is being actively written needs to be analyzed in a programming editor. This error-tolerance ensures tools can keep working even when there are syntax errors, or missing dependencies.
Slang v1’s parser has been focused so far on completeness and correctness. Performance is 4x faster than @solidity-parser/parser, currently being used in many projects across the ecosystem, and we aim to improve on this significantly in the upcoming quarters.
Binding Analysis for symbol tracking
Building tooling often requires understanding code beyond syntax patterns. Identifying semantic meaning to track symbols across code and navigate through it can be essential for many use cases. Slang's binding analysis API allows, for example, to get the list of references to a variable, or to find the definition of a function. It's designed to be error-tolerant as well, providing useful results even with missing dependencies or incomplete code. This makes it perfect for real-world scenarios where you might be working with partial codebases or external dependencies (NPM) that are not available locally. Slang is the only compiler API that offers this capability for Solidity today.
Tree Query Language
Slang brings a CSS selector-like experience to code analysis. Its tree query language makes extracting information from contracts effortless, enabling you to search and filter Solidity code with minimal effort. With a declarative and concise syntax, Slang can replace hundreds or even thousands of lines of code with efficient queries that run on CSTs para preserve every detail.
The usual approach for a very basic extraction of uint
variable definitions is:
function findVariables(node: Node) {
if (node.kind === NonterminalKind.VariableDeclaration) {
// Have to check children manually
for (const child of node.children) {
if (child.kind === NonterminalKind.TypeName) {
// Process type name...
}
if (child.kind === TerminalKind.Identifier) {
// Process identifier...
}
}
}
// Recursively check all children
for (const child of node.children()) {
findVariables(child);
}
}
While Slang makes it a lot less cumbersome:
const query = Query.create(`
[VariableDeclaration
@typeName [TypeName ["uint"]]
@id [Identifier]
]
`);
for (const match of cursor.query([query])) {
const {typeName, id} = match.captures;
// Much easier...
}
The higher the complexity in the code pattern being extracted is, the higher the returns on using Slang’s query language are.
Full-Fidelity Syntax Trees
Slang produces Concrete Syntax Trees (CST) that preserve every detail of code, including comments, whitespace, and formatting. This enables precise tooling that can analyze and transform code while maintaining its original style.
How are Slang’s code analysis APIs being used?
Slang has a handful of early adopters which can be valuable code references for specific use cases:
- Nomic Foundation’s own Solidity VSCode extension, with over 300k downloads, is using it to provide advanced language features like Semantic Highlighting and Document Outline/Symbols. It will be fully migrated to Slang soon.
- The upcoming v2 release of prettier-solidity, enhancing code formatting speed and correctness for tens of thousands of Solidity projects across the ecosystem.
- OpenZeppelin Upgrades uses Slang to analyze the NatSpec comments to implement namespaced storage layout (ERC-7201).
- sspec is a Solidity specification generator for Foundry projects. Using test names it produces human readable descriptions for smart contracts, their functions, and facilitates navigation across tests providing links to functions in test files.
- lintspec is a tool for finding common errors in Solidity documentation. It is a port of natspec-smells to Rust, based on Slang, that is reported to be 214x faster (see announcement).
If you are interested in using Slang in your project, we would love to hear from you! Please reach out to us on our Telegram group, or via GitHub issues.
What's Next on Slang’s Roadmap?
We’ll ship a pragma version extracting API next, and we’ll start working on the backend side to make it possible to build a reliable debugging experience for Solidity within EDR.
Our long-term vision includes building the compiler backend with the same approach of exposing tooling specific APIs. We aim to provide performant, strongly-typed APIs that can be used not only to analyze source code, but also to transform, annotate, validate, and optimize it.
Learn more
- Check out the documentation
- Check out the Github repo.
- Join our Telegram channel.
- Share feedback via GitHub issues.